There are two ways you can play a video. firstly you can embed the .flv video in the .swf file which creates very large and unmanageable files or you can dynamically load the video file which can be done in two ways. First is a streaming video but you will need the Flash Media Server for it or you can have progressive downloaded video like this one which does not need any additional softwares. The actual coding to play a both types of videos is more or less the same
1. First we design the stage. Hit Ctrl+J to bring up the document properties window. Select #333333 as the background color. Now create 2 more layers and name the top most Actions.
2. Hit Ctrl+F8 to bring up the New Symbol window and give the name play_mc. Click on ok and create your own button. Mine looks like this

Now repeat the above process twice and give in the names stop_mc and pause_mc.


make sure atleast the play and pause buttons have exactly the same shape and size. Now select the first frame of the middle layer and drag the play_mc and stop_mc buttons from the library onto the stage and place them near the bottom. Give them instance names of play_mc and stop_mc
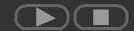
now select the first frame of the bottom most layer and drag the pause button onto it from the library. Give the instance name pause_mc, now from the properties panel set the x and y coordinates of the pause button to be same as that of the play button.Since the pause button is exactly of the same shape and size and is on a lower layer it will be completely hidden.
3.Now again select the first frame of the bottom most layer draw a small line on the side of the buttons with lentgh of 100. make a note of the x and y coordinates. Here its 240 and 385 resp.
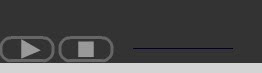
Hit Ctrl+F8 again and this time give the name slider_mc and draw a 16x16 black sqaure and centre it. Go back to the main stage and select the first frame of the middle layer and drag the slider_mc from the library onto the stage and set its coordinates as 340 (240+100) and 385. Give it the instance name of slider_mc in the properties panel
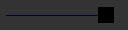
4. Select the Text Tool and draw a dynamic text field near your objects. This will show the duration so place it appropriately. Give it the instance name of dur_txt
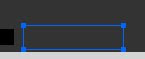
5. now on the bottom most layer above your buttons draw a thin rectangle of length 350 and again make a note of its x and y coordinates. here they are 108 and 357.

Hit Ctrl+F8 again and this time give the name playSeek_mc. Follow the steps as in the volume controller but this time the instance name would be playSeek_mc and the x and y coordinates will be 108 and 360 resp;

6. Now get your .flv file and save it in the same folder as this .swf file and name it myFLV.flv or whatever you want.
7. That done select the first frame of the Actions layer and copy and paste the following code
/*------------------------Video Playback-----------------------------*/
import flash.net.NetConnection;
addEventListener(Event.ENTER_FRAME,trackDuration);
/*several objects have to be declared*/
var videoConnection:NetConnection = new NetConnection();
videoConnection.connect(null);
var videoStream:NetStream = new NetStream(videoConnection);
var video:Video = new Video();
var obj:Object = new Object();
var vDuration=0;/*will contain the total duration of the video*/
/*onMData function called if metadata is detected. Metadata is encoded into
the video by the encoding software*/
obj.onMetaData = onMData;
videoStream.client = obj;
/*Set the dimensions and position of the video*/
video.x=75;
video.y=20
video.width=400;
video.height=300;
addChild(video);/*actually add the video*/
/*all operations will be on the videoStream object*/
video.attachNetStream(videoStream);
function onMData(obj:Object) {
/*Calculate the total duration of the video*/
vDuration=obj.duration;
}
/*denotes whether timeline seekhead is being dragged*/
var isDragging=0;
/*show the duration on stage*/
function trackDuration(e:Event) {
/*videoStream.time give the duration already played*/
dur_txt.text=videoStream.time.toString()
+" / "+vDuration;
/*if seek head not being dragged.This actually helps in smooth dragging
remove this line to see what happens when you drag without this*/
if(isDragging==0)
/*advance the seek head according to duration played
180 is the start position of the seekhead and 350 is
the total lentgh it can be dragged */
playSeek_mc.x = 108+(350*videoStream.time/vDuration);
}
var isPlaying=0;/*denotes if the video is playing*/
var isPaused=0;/*if video is paused*/
play_mc.addEventListener(MouseEvent.CLICK,playHandler);
stop_mc.addEventListener(MouseEvent.CLICK,stopHandler);
pause_mc.addEventListener(MouseEvent.CLICK,pauseHandler);
/*if play button is clicked*/
function playHandler(e:MouseEvent) {
if(isPlaying==0)/*if not already playing*/
{
videoStream.play("myFLV.flv");/*start playing from the beginning*/
isPlaying=1;
}
if(isPaused==1)/*if paused*/
{
videoStream.resume();/*resume playing*/
isPaused=0;
}
e.currentTarget.visible=false;/*hide the play button*/
}
/*if pause button is clicked*/
function pauseHandler(e:MouseEvent) {
videoStream.pause();/*pause play*/
isPaused=1;
play_mc.visible=true;/*show play button*/
}
/*if stop button is clicked*/
function stopHandler(e:MouseEvent) {
videoStream.close();/*stop playing by closing the stream*/
isPlaying=0;
play_mc.visible=true;/*show play button*/
}
/*-------------------------------------------------------------------------------*/
/*------------------------Volume Control-----------------------------------------*/
/*denotes the rectangle boundary in which the volume controller can be dragged
parameters are x,y, width, height. Height=0 since only horizontal movement
is required*/
var rect:Rectangle = new Rectangle(240,385,100,0);
/*--define mouse event handlers for the sliding control---*/
slider_mc.addEventListener(MouseEvent.MOUSE_DOWN,dragStart);
/*notice that the handling function in the following two listeners are the same*/
slider_mc.addEventListener(MouseEvent.MOUSE_UP,dragStop);
slider_mc.addEventListener(MouseEvent.MOUSE_OUT,dragStop);
slider_mc.addEventListener(Event.ENTER_FRAME,adjustVolume);
/*------------------------------------------------------------*/
/*enable dragging if mouse button pressed on it*/
function dragStart(e:MouseEvent) {
slider_mc.startDrag(true,rect);
}
/*stop dragging if moue button released or pointer moved away*/
function dragStop(e:MouseEvent){
slider_mc.stopDrag();
}
function adjustVolume(e:Event) {
/*calculate the volume. 240 is the minimum position and
100 is the width of the drag boundary rectangle "rect"*/
var vol=(slider_mc.x-240)/100;
var volChange:SoundTransform = new SoundTransform(vol);
/*assign the new volume*/
videoStream.soundTransform = volChange;
}
/*--------------------------------------------------------------------------------*/
/*-------------------Timeline Seeking--------------------------------------------*/
/*define mouse event handlers on the timeline seek head*/
playSeek_mc.addEventListener(MouseEvent.MOUSE_DOWN,playDragStart);
playSeek_mc.addEventListener(MouseEvent.MOUSE_UP,playDragStop);
/*denotes the rectangle boundary in which the timeline seek head can be dragged
parameters are x,y, width, height. Height=0 since only horizontal movement
is required*/
var rect1:Rectangle = new Rectangle(108,360,350,0);
/*enable dragging if mouse button pressed on it*/
function playDragStart(e:MouseEvent) {
/*drag only if video is playing and is not paused*/
if(isPlaying==1&&isPaused==0)
{
playSeek_mc.startDrag(true,rect1);
isDragging=1;
}
}
/*stop dragging if moue button released from it*/
function playDragStop(e:MouseEvent){
playSeek_mc.stopDrag();
isDragging=0;
/*determine the position of the seeh head. 108 is the minimum position
350 is the width of the drag boundary rectangle rect1*/
var seekHead=(playSeek_mc.x-108)*vDuration/350;
/*start playing from the position denoted by the seek head*/
videoStream.seek(seekHead);
}
/*-----------------------------------------------------------------------------*/
Now hit Ctrl+Enter and test your movie.
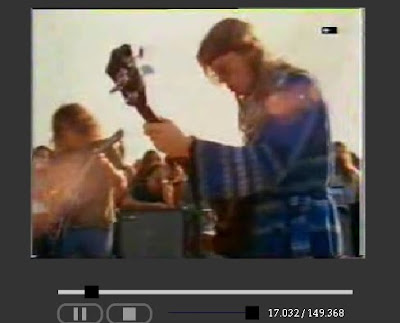
\m/\m/\m/\m/
No comments:
Post a Comment